ex_distributed_tracing_client.c
/*
* This application creates a DT payload, writes it in a request file and
* waits until a response files is created. The creation of a response
* file indicates, that the request file was handled.
*
* The server handling the request is contained in
* ex_distributed_tracing_server.c
*
*/
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include "common.h"
#include "libnewrelic.h"
#define REQUEST_FILE "/tmp/request"
#define RESPONSE_FILE "/tmp/response"
int main(void) {
example_init();
/* Initialize the config */
char* app_name = get_app_name();
if (NULL == app_name) {
return -1;
}
char* license_key = get_license_key();
if (NULL == license_key) {
return -1;
}
newrelic_app_config_t* config
= newrelic_create_app_config(app_name, license_key);
customize_config(&config);
/* Change the transaction tracer threshold to ensure a trace is generated */
/* Enable distributed tracing */
/* Wait up to 10 seconds for the SDK to connect to the daemon */
newrelic_destroy_app_config(&config);
/* Start a web transaction */
/* Start an external segment */
.uri = RESPONSE_FILE});
/* Remove any left over request files */
unlink(REQUEST_FILE);
/*
* Write a request file, which consists of a DT payload.
*
* NOTE: As a convention when communicating by HTTP/HTTPS, applications
* which are instrumented with New Relic send and receive DT
* payloads via the `newrelic` request header.
*/
FILE* request_file = fopen(REQUEST_FILE, "w");
if (request_file) {
printf("Sending a request.\n");
char* payload
= newrelic_create_distributed_trace_payload_httpsafe(txn, segment);
if (payload) {
fprintf(request_file, "%s", payload);
free(payload);
}
fclose(request_file);
/* Wait for a response file to appear */
printf("Waiting for response ... ");
while (true) {
FILE* response_file = fopen(RESPONSE_FILE, "r");
if (response_file) {
printf("received.\n\n");
fclose(response_file);
unlink(RESPONSE_FILE);
break;
}
sleep(1);
}
} else {
"Error.class.request");
printf("Error, cannot send request.\n");
}
/* End the external segment */
newrelic_end_segment(txn, &segment);
/* End web transaction */
newrelic_end_transaction(&txn);
newrelic_destroy_app(&app);
return 0;
}
newrelic_transaction_tracer_threshold_t threshold
Whether to consider transactions for trace generation based on the apdex configuration or a specific ...
Definition: libnewrelic.h:209
struct _newrelic_txn_t newrelic_txn_t
A New Relic transaction.
Definition: libnewrelic.h:106
Definition: libnewrelic.h:191
Segment configuration used to instrument external calls.
Definition: libnewrelic.h:511
char * newrelic_create_distributed_trace_payload_httpsafe(newrelic_txn_t *transaction, newrelic_segment_t *segment)
Create a distributed trace payload, an http-safe, base64-encoded string.
newrelic_txn_t * newrelic_start_web_transaction(newrelic_app_t *app, const char *name)
Start a web based transaction.
Configuration used to describe application name, license key, as well as optional transaction tracer ...
Definition: libnewrelic.h:347
struct _nr_app_and_info_t newrelic_app_t
A New Relic application. Once an application configuration is created with newrelic_create_app_config...
Definition: libnewrelic.h:95
bool newrelic_end_segment(newrelic_txn_t *transaction, newrelic_segment_t **segment_ptr)
Record the completion of a segment in a transaction.
newrelic_transaction_tracer_config_t transaction_tracer
Optional. The transaction tracer configuration.
Definition: libnewrelic.h:390
newrelic_app_config_t * newrelic_create_app_config(const char *app_name, const char *license_key)
Create a populated application configuration.
newrelic_app_t * newrelic_create_app(const newrelic_app_config_t *config, unsigned short timeout_ms)
Create an application.
newrelic_segment_t * newrelic_start_external_segment(newrelic_txn_t *transaction, const newrelic_external_segment_params_t *params)
Start recording an external segment within a transaction.
newrelic_time_us_t duration_us
If the SDK configuration threshold is set to NEWRELIC_THRESHOLD_IS_OVER_DURATION, this field specifie...
Definition: libnewrelic.h:218
newrelic_distributed_tracing_config_t distributed_tracing
Optional. Distributed tracing configuration.
Definition: libnewrelic.h:408
bool newrelic_destroy_app_config(newrelic_app_config_t **config)
Destroy the application configuration.
struct _newrelic_segment_t newrelic_segment_t
A segment within a transaction.
Definition: libnewrelic.h:840
bool newrelic_end_transaction(newrelic_txn_t **transaction_ptr)
End a transaction.
This is the New Relic C SDK! If your application does not use other New Relic APM agent languages,...
void newrelic_notice_error(newrelic_txn_t *transaction, int priority, const char *errmsg, const char *errclass)
Record an error in a transaction.
bool enabled
Specifies whether or not distributed tracing is enabled.
Definition: libnewrelic.h:323
Generated on Wed Jan 8 2020 01:11:45 for New Relic C SDK by
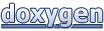