This is the New Relic C SDK! If your application does not use other New Relic APM agent languages, you can use the C SDK to take advantage of New Relic's monitoring capabilities and features to instrument a wide range of applications. More...
Go to the source code of this file.
Data Structures | |
struct | newrelic_transaction_tracer_config_t |
Configuration used to configure transaction tracing. More... | |
struct | newrelic_datastore_segment_config_t |
Configuration used to configure how datastore segments are recorded in a transaction. More... | |
struct | newrelic_distributed_tracing_config_t |
Configuration used for distributed tracing. More... | |
struct | newrelic_span_event_config_t |
Configuration used for span events. More... | |
struct | newrelic_app_config_t |
Configuration used to describe application name, license key, as well as optional transaction tracer and datastore configuration. More... | |
struct | newrelic_datastore_segment_params_t |
Segment configuration used to instrument calls to databases and object stores. More... | |
struct | newrelic_external_segment_params_t |
Segment configuration used to instrument external calls. More... | |
Macros | |
Datastore product names with query instrumentation support | |
The product field of the newrelic_datastore_segment_params_t specifies the datastore type of a datastore segment. For example, "MySQL" indicates that the segment's query is against a MySQL database. New Relic recommends using the predefined NEWRELIC_DATASTORE_FIREBIRD through NEWRELIC_DATASTORE_SYBASE constants for this field. For the SQL-like datastores which are supported by the C SDK, when the record_sql field of the newrelic_transaction_tracer_config_t is set to NEWRELIC_SQL_RAW or NEWRELIC_SQL_OBFUSCATED, the query param of the newrelic_datastore_segment_config_t is reported to New Relic. | |
#define | NEWRELIC_DATASTORE_FIREBIRD "Firebird" |
#define | NEWRELIC_DATASTORE_INFORMIX "Informix" |
#define | NEWRELIC_DATASTORE_MSSQL "MSSQL" |
#define | NEWRELIC_DATASTORE_MYSQL "MySQL" |
#define | NEWRELIC_DATASTORE_ORACLE "Oracle" |
#define | NEWRELIC_DATASTORE_POSTGRES "Postgres" |
#define | NEWRELIC_DATASTORE_SQLITE "SQLite" |
#define | NEWRELIC_DATASTORE_SYBASE "Sybase" |
Datastore product names without query instrumentation support | |
The product field of newrelic_datastore_segment_params_t specifies the datastore type of a datastore segment. If the product field points to a string that is one of NEWRELIC_DATASTORE_MEMCACHE through NEWRELIC_DATASTORE_OTHER, the resulting datastore segment shall be instrumented as an unsupported datastore; this datastore's query language has no obfuscation support and its query cannot securely be reported to New Relic. | |
#define | NEWRELIC_DATASTORE_MEMCACHE "Memcached" |
#define | NEWRELIC_DATASTORE_MONGODB "MongoDB" |
#define | NEWRELIC_DATASTORE_ODBC "ODBC" |
#define | NEWRELIC_DATASTORE_REDIS "Redis" |
#define | NEWRELIC_DATASTORE_OTHER "Other" |
Transport types for distributed tracing instrumentation | |
The function newrelic_accept_distributed_trace_payload() accepts a transport type which represents the type of connection used to transmit the trace payload. These transport names are used across New Relic agents and are used by the UI to group traces. | |
#define | NEWRELIC_TRANSPORT_TYPE_UNKNOWN "Unknown" |
#define | NEWRELIC_TRANSPORT_TYPE_HTTP "HTTP" |
#define | NEWRELIC_TRANSPORT_TYPE_HTTPS "HTTPS" |
#define | NEWRELIC_TRANSPORT_TYPE_KAFKA "Kafka" |
#define | NEWRELIC_TRANSPORT_TYPE_JMS "JMS" |
#define | NEWRELIC_TRANSPORT_TYPE_IRONMQ "IronMQ" |
#define | NEWRELIC_TRANSPORT_TYPE_AMQP "AMQP" |
#define | NEWRELIC_TRANSPORT_TYPE_QUEUE "Queue" |
#define | NEWRELIC_TRANSPORT_TYPE_OTHER "Other" |
Typedefs | |
typedef struct _nr_app_and_info_t | newrelic_app_t |
A New Relic application. Once an application configuration is created with newrelic_create_app_config(), call newrelic_create_app() to create an application to report data to the daemon; the daemon, in turn, reports data to New Relic. More... | |
typedef struct _newrelic_txn_t | newrelic_txn_t |
A New Relic transaction. More... | |
typedef uint64_t | newrelic_time_us_t |
A time, measured in microseconds. More... | |
typedef struct _newrelic_segment_t | newrelic_segment_t |
A segment within a transaction. More... | |
typedef struct _newrelic_custom_event_t | newrelic_custom_event_t |
A Custom Event. More... | |
Enumerations | |
enum | newrelic_loglevel_t { NEWRELIC_LOG_ERROR, NEWRELIC_LOG_WARNING, NEWRELIC_LOG_INFO, NEWRELIC_LOG_DEBUG } |
Log levels. More... | |
enum | newrelic_tt_recordsql_t { NEWRELIC_SQL_OFF, NEWRELIC_SQL_RAW, NEWRELIC_SQL_OBFUSCATED } |
Configuration values used to configure how SQL queries are recorded and reported to New Relic. More... | |
enum | newrelic_transaction_tracer_threshold_t { NEWRELIC_THRESHOLD_IS_APDEX_FAILING, NEWRELIC_THRESHOLD_IS_OVER_DURATION } |
Configuration values used to configure the behaviour of the transaction tracer. More... | |
Functions | |
bool | newrelic_configure_log (const char *filename, newrelic_loglevel_t level) |
Configure the C SDK's logging system. More... | |
bool | newrelic_init (const char *daemon_socket, int time_limit_ms) |
Initialise the C SDK with non-default settings. More... | |
newrelic_app_config_t * | newrelic_create_app_config (const char *app_name, const char *license_key) |
Create a populated application configuration. More... | |
bool | newrelic_destroy_app_config (newrelic_app_config_t **config) |
Destroy the application configuration. More... | |
newrelic_app_t * | newrelic_create_app (const newrelic_app_config_t *config, unsigned short timeout_ms) |
Create an application. More... | |
bool | newrelic_destroy_app (newrelic_app_t **app) |
Destroy the application. More... | |
newrelic_txn_t * | newrelic_start_web_transaction (newrelic_app_t *app, const char *name) |
Start a web based transaction. More... | |
newrelic_txn_t * | newrelic_start_non_web_transaction (newrelic_app_t *app, const char *name) |
Start a non-web based transaction. More... | |
bool | newrelic_set_transaction_timing (newrelic_txn_t *transaction, newrelic_time_us_t start_time, newrelic_time_us_t duration) |
Override the timing for the given transaction. More... | |
bool | newrelic_end_transaction (newrelic_txn_t **transaction_ptr) |
End a transaction. More... | |
bool | newrelic_add_attribute_int (newrelic_txn_t *transaction, const char *key, const int value) |
Add a custom integer attribute to a transaction. More... | |
bool | newrelic_add_attribute_long (newrelic_txn_t *transaction, const char *key, const long value) |
Add a custom long attribute to a transaction. More... | |
bool | newrelic_add_attribute_double (newrelic_txn_t *transaction, const char *key, const double value) |
Add a custom double attribute to a transaction. More... | |
bool | newrelic_add_attribute_string (newrelic_txn_t *transaction, const char *key, const char *value) |
Add a custom string attribute to a transaction. More... | |
void | newrelic_notice_error (newrelic_txn_t *transaction, int priority, const char *errmsg, const char *errclass) |
Record an error in a transaction. More... | |
newrelic_segment_t * | newrelic_start_segment (newrelic_txn_t *transaction, const char *name, const char *category) |
Record the start of a custom segment in a transaction. More... | |
newrelic_segment_t * | newrelic_start_datastore_segment (newrelic_txn_t *transaction, const newrelic_datastore_segment_params_t *params) |
Record the start of a datastore segment in a transaction. More... | |
newrelic_segment_t * | newrelic_start_external_segment (newrelic_txn_t *transaction, const newrelic_external_segment_params_t *params) |
Start recording an external segment within a transaction. More... | |
bool | newrelic_set_segment_parent (newrelic_segment_t *segment, newrelic_segment_t *parent) |
Set the parent for the given segment. More... | |
bool | newrelic_set_segment_parent_root (newrelic_segment_t *segment) |
Set the transaction's root as the parent for the given segment. More... | |
bool | newrelic_set_segment_timing (newrelic_segment_t *segment, newrelic_time_us_t start_time, newrelic_time_us_t duration) |
Override the timing for the given segment. More... | |
bool | newrelic_end_segment (newrelic_txn_t *transaction, newrelic_segment_t **segment_ptr) |
Record the completion of a segment in a transaction. More... | |
newrelic_custom_event_t * | newrelic_create_custom_event (const char *event_type) |
Creates a custom event. More... | |
void | newrelic_discard_custom_event (newrelic_custom_event_t **event) |
Frees the memory for custom events created via the newrelic_create_custom_event function. More... | |
void | newrelic_record_custom_event (newrelic_txn_t *transaction, newrelic_custom_event_t **event) |
Records the custom event. More... | |
bool | newrelic_custom_event_add_attribute_int (newrelic_custom_event_t *event, const char *key, int value) |
Adds an int key/value pair to the custom event's attributes. More... | |
bool | newrelic_custom_event_add_attribute_long (newrelic_custom_event_t *event, const char *key, long value) |
Adds a long key/value pair to the custom event's attributes. More... | |
bool | newrelic_custom_event_add_attribute_double (newrelic_custom_event_t *event, const char *key, double value) |
Adds a double key/value pair to the custom event's attributes. More... | |
bool | newrelic_custom_event_add_attribute_string (newrelic_custom_event_t *event, const char *key, const char *value) |
Adds a string key/value pair to the custom event's attributes. More... | |
const char * | newrelic_version (void) |
Get the SDK version. More... | |
bool | newrelic_record_custom_metric (newrelic_txn_t *transaction, const char *metric_name, double milliseconds) |
Generate a custom metric. More... | |
bool | newrelic_ignore_transaction (newrelic_txn_t *transaction) |
Ignore the current transaction. More... | |
char * | newrelic_create_distributed_trace_payload (newrelic_txn_t *transaction, newrelic_segment_t *segment) |
Create a distributed trace payload. More... | |
bool | newrelic_accept_distributed_trace_payload (newrelic_txn_t *transaction, const char *payload, const char *transport_type) |
Accept a distributed trace payload. More... | |
char * | newrelic_create_distributed_trace_payload_httpsafe (newrelic_txn_t *transaction, newrelic_segment_t *segment) |
Create a distributed trace payload, an http-safe, base64-encoded string. More... | |
bool | newrelic_accept_distributed_trace_payload_httpsafe (newrelic_txn_t *transaction, const char *payload, const char *transport_type) |
Accept a distributed trace payload, an http-safe, base64-encoded string. More... | |
bool | newrelic_set_transaction_name (newrelic_txn_t *transaction, const char *transaction_name) |
Set a transaction name. More... | |
Detailed Description
This is the New Relic C SDK! If your application does not use other New Relic APM agent languages, you can use the C SDK to take advantage of New Relic's monitoring capabilities and features to instrument a wide range of applications.
See accompanying GUIDE.md and LICENSE.txt for more information.
Typedef Documentation
◆ newrelic_app_t
typedef struct _nr_app_and_info_t newrelic_app_t |
A New Relic application. Once an application configuration is created with newrelic_create_app_config(), call newrelic_create_app() to create an application to report data to the daemon; the daemon, in turn, reports data to New Relic.
- See also
- newrelic_create_app().
◆ newrelic_custom_event_t
typedef struct _newrelic_custom_event_t newrelic_custom_event_t |
A Custom Event.
The C SDK provides a Custom Events API that allows one to send custom events to New Relic Insights. To send an event, start a transaction and use the newrelic_create_custom_event() and newrelic_record_custom_event() functions. Examples of sending custom events are available in the examples directory.
◆ newrelic_segment_t
typedef struct _newrelic_segment_t newrelic_segment_t |
A segment within a transaction.
Within an active transaction, instrument additional segments for greater granularity.
-For external calls: newrelic_start_external_segment(). -For datastore calls: newrelic_start_datastore_segment(). -For arbitrary code: newrelic_start_segment().
All segments must be ended with newrelic_end_segment(). Examples of instrumenting segments are available in the examples directory.
◆ newrelic_time_us_t
typedef uint64_t newrelic_time_us_t |
A time, measured in microseconds.
C SDK configuration and API calls with time-related parameters expect this type. For an example configuration, see newrelic_transaction_tracer_config_t.
◆ newrelic_txn_t
typedef struct _newrelic_txn_t newrelic_txn_t |
A New Relic transaction.
A transaction is started using newrelic_start_web_transaction() or newrelic_start_non_web_transaction(). A started, or active, transaction is stopped using newrelic_end_transaction(). One may modify a transaction by adding custom attributes or recording errors only after it has been started.
Enumeration Type Documentation
◆ newrelic_loglevel_t
enum newrelic_loglevel_t |
Log levels.
An enumeration of the possible log levels for an SDK configuration, or newrelic_app_config_t. The highest priority loglevel is NEWRELIC_LOG_ERROR. The level NEWRELIC_LOG_DEBUG offers the greatest verbosity.
- See also
- newrelic_app_config_t
◆ newrelic_transaction_tracer_threshold_t
Configuration values used to configure the behaviour of the transaction tracer.
◆ newrelic_tt_recordsql_t
Configuration values used to configure how SQL queries are recorded and reported to New Relic.
Enumerator | |
---|---|
NEWRELIC_SQL_OFF | When the record_sql field of the newrelic_transaction_tracer_config_t is set to NEWRELIC_SQL_OFF, no queries are reported to New Relic. |
NEWRELIC_SQL_RAW | For the SQL-like datastores which are supported by the C SDK, when the record_sql field of the newrelic_transaction_tracer_config_t is set to NEWRELIC_SQL_RAW the query param of the newrelic_datastore_segment_config_t is reported as-is to New Relic.
|
NEWRELIC_SQL_OBFUSCATED | For the SQL-like datastores which are supported by the C SDK, when the record_sql field of the newrelic_transaction_tracer_config_t is set to NEWRELIC_SQL_RAW the query param of the newrelic_datastore_segment_config_t is reported to New Relic with alphanumeric characters set to '?'. |
Function Documentation
◆ newrelic_accept_distributed_trace_payload()
bool newrelic_accept_distributed_trace_payload | ( | newrelic_txn_t * | transaction, |
const char * | payload, | ||
const char * | transport_type | ||
) |
Accept a distributed trace payload.
Accept newrelic headers, or a payload, created with newrelic_create_distributed_trace_payload(). Such headers are manually added to a service's outbound request. The receiving service gets the newrelic header from the incoming request and uses this function to accept the payload and link corresponding spans together for a complete distributed trace. Note that a payload must be accepted within an active transaction.
- Parameters
-
[in] transaction An active transaction. This value cannot be NULL. [in] payload A string created by newrelic_create_distributed_trace_payload(). This value cannot be NULL. [in] transport_type Transport type used for communicating the external call. It is strongly recommended that one of NEWRELIC_TRANSPORT_TYPE_UNKNOWN through NEWRELIC_TRANSPORT_TYPE_OTHER are used for this value. If NULL is supplied for this parameter, an info-level message is logged and the default value of NEWRELIC_TRANSPORT_TYPE_UNKNOWN is used.
- Returns
- true on success.
◆ newrelic_accept_distributed_trace_payload_httpsafe()
bool newrelic_accept_distributed_trace_payload_httpsafe | ( | newrelic_txn_t * | transaction, |
const char * | payload, | ||
const char * | transport_type | ||
) |
Accept a distributed trace payload, an http-safe, base64-encoded string.
This function offers the same behaviour as newrelic_accept_distributed_trace_payload() but accepts a base64-encoded string for the payload.
- Examples
- ex_distributed_tracing_server.c.
◆ newrelic_add_attribute_double()
bool newrelic_add_attribute_double | ( | newrelic_txn_t * | transaction, |
const char * | key, | ||
const double | value | ||
) |
Add a custom double attribute to a transaction.
Given an active transaction, this function appends a double attribute to the transaction.
- Parameters
-
[in] transaction An active transaction. [in] key The name of the attribute. [in] value The double value of the attribute.
- Returns
- true if successful; false otherwise.
◆ newrelic_add_attribute_int()
bool newrelic_add_attribute_int | ( | newrelic_txn_t * | transaction, |
const char * | key, | ||
const int | value | ||
) |
Add a custom integer attribute to a transaction.
Given an active transaction, this function appends an integer attribute to the transaction.
- Parameters
-
[in] transaction An active transaction. [in] key The name of the attribute. [in] value The integer value of the attribute.
- Returns
- true if successful; false otherwise.
- Examples
- ex_notice_error.c.
◆ newrelic_add_attribute_long()
bool newrelic_add_attribute_long | ( | newrelic_txn_t * | transaction, |
const char * | key, | ||
const long | value | ||
) |
Add a custom long attribute to a transaction.
Given an active transaction, this function appends a long attribute to the transaction.
- Parameters
-
[in] transaction An active transaction. [in] key The name of the attribute. [in] value The long value of the attribute.
- Returns
- true if successful; false otherwise.
◆ newrelic_add_attribute_string()
bool newrelic_add_attribute_string | ( | newrelic_txn_t * | transaction, |
const char * | key, | ||
const char * | value | ||
) |
Add a custom string attribute to a transaction.
Given an active transaction, this function appends a string attribute to the transaction.
- Parameters
-
[in] transaction An active transaction. [in] key The name of the attribute. [in] value The string value of the attribute.
- Returns
- true if successful; false otherwise.
◆ newrelic_configure_log()
bool newrelic_configure_log | ( | const char * | filename, |
newrelic_loglevel_t | level | ||
) |
Configure the C SDK's logging system.
If the logging system was previously initialized (either by a prior call to newrelic_configure_log() or implicitly by a call to newrelic_init() or newrelic_create_app()), then invoking this function will close the previous log file.
- Parameters
-
[in] filename The path to the file to write logs to. If this is the literal string "stdout" or "stderr", then logs will be written to standard output or standard error, respectively. [in] level The lowest level of log message that will be output.
- Returns
- true on success; false otherwise.
- Examples
- ex_simple.c.
◆ newrelic_create_app()
newrelic_app_t* newrelic_create_app | ( | const newrelic_app_config_t * | config, |
unsigned short | timeout_ms | ||
) |
Create an application.
Given a configuration, newrelic_create_app() returns a pointer to the newly allocated application, or NULL if there was an error. If successful, the caller should destroy the application with the supplied newrelic_destroy_app() when finished.
- Parameters
-
[in] config An application configuration created by newrelic_create_app_config(). [in] timeout_ms Specifies the maximum time to wait for a connection to be established; a value of 0 causes the method to make only one attempt at connecting to the daemon.
- Returns
- A pointer to an allocated application, or NULL on error; any errors resulting from a badly-formed configuration are logged.
◆ newrelic_create_app_config()
newrelic_app_config_t* newrelic_create_app_config | ( | const char * | app_name, |
const char * | license_key | ||
) |
Create a populated application configuration.
Given an application name and license key, this method returns an SDK configuration. Specifically, it returns a pointer to a newrelic_app_config_t with initialized app_name and license_key fields along with default values for the remaining fields. After the application has been created with newrelic_create_app(), the caller should free the configuration using newrelic_destroy_app_config().
- Parameters
-
[in] app_name The name of the application. [in] license_key A valid license key supplied by New Relic.
- Returns
- An application configuration populated with app_name and license_key; all other fields are initialized to their defaults.
◆ newrelic_create_custom_event()
newrelic_custom_event_t* newrelic_create_custom_event | ( | const char * | event_type | ) |
Creates a custom event.
Attributes can be added to the custom event using the newrelic_custom_event_add_* family of functions. When the required attributes have been added, the custom event can be recorded using newrelic_record_custom_event().
When passed to newrelic_record_custom_event, the custom event will be freed. If you can't pass an allocated event to newrelic_record_custom_event, use the newrelic_discard_custom_event function to free the event.
- Parameters
-
[in] event_type The type/name of the event
- Returns
- A pointer to a custom event; NULL otherwise.
- Examples
- ex_custom.c.
◆ newrelic_create_distributed_trace_payload()
char* newrelic_create_distributed_trace_payload | ( | newrelic_txn_t * | transaction, |
newrelic_segment_t * | segment | ||
) |
Create a distributed trace payload.
Create a newrelic header, or a payload, to add to a service's outbound requests. This header contains the metadata necessary to link spans together for a complete distributed trace. The metadata includes: the trace ID number, the span ID number, New Relic account ID number, and sampling information. Note that a payload must be created within an active transaction.
- Parameters
-
[in] transaction An active transaction. This value cannot be NULL. [in] segment An active segment in which the distributed trace payload is being created, or NULL to indicate that the payload is created for the root segment.
- Returns
- If successful, a string to manually add to a service's outbound requests. If the instrumented application has not established a connection to the daemon or if distributed tracing is not enabled in the newrelic_app_config_t, this function returns NULL. The caller is responsible for invoking free() on the returned string.
◆ newrelic_create_distributed_trace_payload_httpsafe()
char* newrelic_create_distributed_trace_payload_httpsafe | ( | newrelic_txn_t * | transaction, |
newrelic_segment_t * | segment | ||
) |
Create a distributed trace payload, an http-safe, base64-encoded string.
This function offers the same behaviour as newrelic_create_distributed_trace_payload() but creates a base64-encoded string for the payload. The caller is responsible for invoking free() on the returned string.
- Examples
- ex_distributed_tracing_client.c.
◆ newrelic_custom_event_add_attribute_double()
bool newrelic_custom_event_add_attribute_double | ( | newrelic_custom_event_t * | event, |
const char * | key, | ||
double | value | ||
) |
Adds a double key/value pair to the custom event's attributes.
Given a custom event, this function adds a double attribute to the event.
- Parameters
-
[in] event A valid custom event created by newrelic_create_custom_event() [in] key the string key for the key/value pair [in] value the double value of the key/value pair
- Returns
- false indicates the attribute could not be added
- Examples
- ex_custom.c.
◆ newrelic_custom_event_add_attribute_int()
bool newrelic_custom_event_add_attribute_int | ( | newrelic_custom_event_t * | event, |
const char * | key, | ||
int | value | ||
) |
Adds an int key/value pair to the custom event's attributes.
Given a custom event, this function adds an integer attributes to the event.
- Parameters
-
[in] event A valid custom event,
- See also
- newrelic_create_custom_event()
- Parameters
-
[in] key the string key for the key/value pair [in] value the integer value of the key/value pair
- Returns
- false indicates the attribute could not be added
- Examples
- ex_custom.c.
◆ newrelic_custom_event_add_attribute_long()
bool newrelic_custom_event_add_attribute_long | ( | newrelic_custom_event_t * | event, |
const char * | key, | ||
long | value | ||
) |
Adds a long key/value pair to the custom event's attributes.
Given a custom event, this function adds a long attribute to the event.
- Parameters
-
[in] event A valid custom event created by newrelic_create_custom_event() [in] key the string key for the key/value pair [in] value the long value of the key/value pair
- Returns
- false indicates the attribute could not be added
- Examples
- ex_custom.c.
◆ newrelic_custom_event_add_attribute_string()
bool newrelic_custom_event_add_attribute_string | ( | newrelic_custom_event_t * | event, |
const char * | key, | ||
const char * | value | ||
) |
Adds a string key/value pair to the custom event's attributes.
Given a custom event, this function adds a char* (string) attribute to the event.
- Parameters
-
[in] event A valid custom event created by newrelic_create_custom_event(). [in] key the string key for the key/value pair [in] value the string value of the key/value pair
- Returns
- false indicates the attribute could not be added
- Examples
- ex_custom.c.
◆ newrelic_destroy_app()
bool newrelic_destroy_app | ( | newrelic_app_t ** | app | ) |
Destroy the application.
Given an allocated application, newrelic_destroy_app() closes the logfile handle and frees any memory used by app to describe the application.
- Parameters
-
[in] app The address of the pointer to the allocated application.
- Returns
- false if app is NULL or points to NULL; true otherwise.
- Warning
- This function must only be called once for a given application.
◆ newrelic_destroy_app_config()
bool newrelic_destroy_app_config | ( | newrelic_app_config_t ** | config | ) |
Destroy the application configuration.
Given an allocated application configuration, newrelic_destroy_app_config() frees the configuration.
- Parameters
-
[in] config The address of the pointer to the allocated application configuration.
- Returns
- false if config is NULL or points to NULL; true otherwise.
- Warning
- This function must only be called once for a given application configuration.
◆ newrelic_discard_custom_event()
void newrelic_discard_custom_event | ( | newrelic_custom_event_t ** | event | ) |
Frees the memory for custom events created via the newrelic_create_custom_event function.
This function is here in case there's an allocated newrelic_custom_event_t that ends up not being recorded as a custom event, but still needs to be freed
- Parameters
-
[in] event The address of a valid custom event created by newrelic_create_custom_event().
◆ newrelic_end_segment()
bool newrelic_end_segment | ( | newrelic_txn_t * | transaction, |
newrelic_segment_t ** | segment_ptr | ||
) |
Record the completion of a segment in a transaction.
Given an active transaction, this function records the segment's metrics on the transaction.
- Parameters
-
[in] transaction An active transaction. [in,out] segment_ptr The address of a valid segment. Before the function returns, any segment_ptr memory is freed; segment_ptr is set to NULL to avoid any potential double free errors.
- Returns
- true if the parameters represented an active transaction and custom segment to record as complete; false otherwise. If an error occurred, a log message will be written to the SDK log at LOG_ERROR level.
◆ newrelic_end_transaction()
bool newrelic_end_transaction | ( | newrelic_txn_t ** | transaction_ptr | ) |
End a transaction.
Given an active transaction, this function stops the transaction's timing, sends any data to the New Relic daemon, and destroys the transaction.
- Parameters
-
[in] transaction_ptr The address of a pointer to an active transaction.
- Returns
- false if transaction is NULL or points to NULL; false if data cannot be sent to newrelic; true otherwise.
- Warning
- This function must only be called once for a given transaction.
◆ newrelic_ignore_transaction()
bool newrelic_ignore_transaction | ( | newrelic_txn_t * | transaction | ) |
Ignore the current transaction.
Given a transaction, this function instructs the C SDK to not send data to New Relic for that transaction.
- Warning
- Even when newrelic_ignore_transaction() is called, one must still call newrelic_end_transaction() to free the memory used by the transaction and avoid a memory leak.
- Parameters
-
[in] transaction An active transaction.
- Returns
- true on success.
◆ newrelic_init()
bool newrelic_init | ( | const char * | daemon_socket, |
int | time_limit_ms | ||
) |
Initialise the C SDK with non-default settings.
Generally, this function only needs to be called explicitly if the daemon socket location needs to be customised. By default, "/tmp/.newrelic.sock" is used, which matches the default socket location used by newrelic-daemon if one isn't given.
The daemon socket location can be specified in four different ways:
- To use a specified file as a UNIX domain socket (UDS), provide an absolute path name as a string.
- To use a standard TCP port, specify a number in the range 1 to 65534.
- To use an abstract socket, prefix the socket name with '@'.
- To connect to a daemon that is running on a different host, set this value to '<host>:<port>', where '<host>' denotes either a host name or an IP address, and '<port>' denotes a valid port number. Both IPv4 and IPv6 are supported.
If an explicit call to this function is required, it must occur before the first call to newrelic_create_app().
Subsequent calls to this function after a successful call to newrelic_init() or newrelic_create_app() will fail.
- Parameters
-
[in] daemon_socket The path to the daemon socket. If this is NULL, then the default will be used, which is to look for a UNIX domain socket at /tmp/.newrelic.sock. [in] time_limit_ms The amount of time, in milliseconds, that the C SDK will wait for a response from the daemon before considering initialization to have failed. If this is 0, then a default value will be used.
- Returns
- true on success; false otherwise.
- Examples
- ex_simple.c.
◆ newrelic_notice_error()
void newrelic_notice_error | ( | newrelic_txn_t * | transaction, |
int | priority, | ||
const char * | errmsg, | ||
const char * | errclass | ||
) |
Record an error in a transaction.
Given an active transaction, this function records an error inside of the transaction.
- Parameters
-
[in] transaction An active transaction. [in] priority The error's priority. The C SDK sends up one error per transaction. If multiple calls to this function are made during a single transaction, the error with the highest priority is reported to New Relic. [in] errmsg A string comprising the error message. [in] errclass A string comprising the error class.
◆ newrelic_record_custom_event()
void newrelic_record_custom_event | ( | newrelic_txn_t * | transaction, |
newrelic_custom_event_t ** | event | ||
) |
Records the custom event.
Given an active transaction, this function adds the custom event to the transaction and timestamps it, ensuring the event will be sent to New Relic.
- Parameters
-
[in] transaction An active transaction [in] event The address of a valid custom event created by newrelic_create_custom_event()
newrelic_create_custom_event
- Examples
- ex_custom.c.
◆ newrelic_record_custom_metric()
bool newrelic_record_custom_metric | ( | newrelic_txn_t * | transaction, |
const char * | metric_name, | ||
double | milliseconds | ||
) |
Generate a custom metric.
Given an active transaction and valid parameters, this function creates a custom metric to be recorded as part of the transaction.
- Parameters
-
[in] transaction An active transaction. [in] metric_name The name/identifier for the metric. [in] milliseconds The amount of time the metric will record, in milliseconds.
- Returns
- true on success.
- Examples
- ex_custom.c.
◆ newrelic_set_segment_parent()
bool newrelic_set_segment_parent | ( | newrelic_segment_t * | segment, |
newrelic_segment_t * | parent | ||
) |
Set the parent for the given segment.
This function changes the parent for the given segment to another segment. Both segments must exist on the same transaction, and must not have ended.
- Parameters
-
[in] segment The segment to reparent. [in] parent The new parent segment.
- Returns
- true if the segment was successfully reparented; false otherwise.
- Warning
- Do not attempt to use a segment that has had newrelic_end_segment() called on it as a segment or parent: this will result in a use-after-free scenario, and likely a crash.
- Examples
- ex_segment.c.
◆ newrelic_set_segment_parent_root()
bool newrelic_set_segment_parent_root | ( | newrelic_segment_t * | segment | ) |
Set the transaction's root as the parent for the given segment.
Transactions are represented by a collection of segments. Segments are created by calls to newrelic_start_segment(), newrelic_start_datastore_segment() and newrelic_start_external_segment(). In addition, a transaction has an automatically-created root segment that represents the entrypoint of the transaction. In some cases, users may want to manually parent their segments with the transaction's root segment.
- Parameters
-
[in] segment The segment to be parented.
- Returns
- true if the segment was successfully reparented; false otherwise.
- Warning
- Do not attempt to use a segment that has had newrelic_end_segment() called on it as a segment or parent: this will result in a use-after-free scenario, and likely a crash.
- Examples
- ex_segment.c.
◆ newrelic_set_segment_timing()
bool newrelic_set_segment_timing | ( | newrelic_segment_t * | segment, |
newrelic_time_us_t | start_time, | ||
newrelic_time_us_t | duration | ||
) |
Override the timing for the given segment.
Segments are normally timed automatically based on when they were started and ended. Calling this function disables the automatic timing, and uses the times given instead.
Note that this may cause unusual looking transaction traces, as this function does not change the parent segment. It is likely that users of this function will also want to use newrelic_set_segment_parent() to manually parent their segments.
- Parameters
-
[in] segment The segment to manually time. [in] start_time The start time for the segment, in microseconds since the start of the transaction. [in] duration The duration of the segment in microseconds.
- Returns
- true if the segment timing was changed; false otherwise.
- Examples
- ex_segment.c, and ex_timing.c.
◆ newrelic_set_transaction_name()
bool newrelic_set_transaction_name | ( | newrelic_txn_t * | transaction, |
const char * | transaction_name | ||
) |
Set a transaction name.
Given an active transaction and a name, this function sets the transaction name to the given name.
- Parameters
-
[in] transaction An active transaction. [in] name Name for the transaction.
- Returns
- true on success.
- Warning
- Do not use brackets [] at the end of your transaction name. New Relic automatically strips brackets from the name. Instead, use parentheses () or other symbols if needed.
◆ newrelic_set_transaction_timing()
bool newrelic_set_transaction_timing | ( | newrelic_txn_t * | transaction, |
newrelic_time_us_t | start_time, | ||
newrelic_time_us_t | duration | ||
) |
Override the timing for the given transaction.
Transactions are normally timed automatically based on when they were started and ended. Calling this function disables the automatic timing, and uses the times given instead.
Note that this may cause unusual looking transaction traces. This function manually alters a transaction's start time and duration, but it does not alter any timing for the segments belonging to the transaction. As a result, the sum of all segment durations may be substantively greater or less than the total duration of the transaction.
It is likely that users of this function will also want to use newrelic_set_segment_timing() to manually time their segments.
- Parameters
-
[in] transaction The transaction to manually time. [in] start_time The start time for the segment, in microseconds since the UNIX Epoch. [in] duration The duration of the transaction in microseconds.
- Returns
- true if the segment timing was changed; false otherwise.
- Examples
- ex_segment.c, and ex_timing.c.
◆ newrelic_start_datastore_segment()
newrelic_segment_t* newrelic_start_datastore_segment | ( | newrelic_txn_t * | transaction, |
const newrelic_datastore_segment_params_t * | params | ||
) |
Record the start of a datastore segment in a transaction.
Given an active transaction and valid parameters, this function creates a datastore segment to be recorded as part of the transaction. A subsequent call to newrelic_end_segment() records the end of the segment.
- Parameters
-
[in] transaction An active transaction. [in] params Valid parameters describing a datastore segment.
- Returns
- A pointer to a valid datastore segment; NULL otherwise.
- Examples
- ex_datastore.c.
◆ newrelic_start_external_segment()
newrelic_segment_t* newrelic_start_external_segment | ( | newrelic_txn_t * | transaction, |
const newrelic_external_segment_params_t * | params | ||
) |
Start recording an external segment within a transaction.
Given an active transaction, this function creates an external segment inside of the transaction and marks it as having been started. An external segment is generally used to represent a HTTP or RPC request.
- Parameters
-
[in] transaction An active transaction. [in] params The parameters describing the external request. All parameters are copied, and no references to the pointers provided are kept after this function returns.
- Returns
- A pointer to an external segment, which may then be provided to newrelic_end_segment() when the external request is complete. If an error occurs when creating the external segment, NULL is returned, and a log message will be written to the SDK log at LOG_ERROR level.
- Examples
- ex_distributed_tracing_client.c, and ex_external.c.
◆ newrelic_start_non_web_transaction()
newrelic_txn_t* newrelic_start_non_web_transaction | ( | newrelic_app_t * | app, |
const char * | name | ||
) |
Start a non-web based transaction.
Given a valid application and transaction name, this function begins timing a new transaction and returns a valid pointer to a New Relic transaction, newrelic_txn_t. The return value of this function may be used as an input parameter to functions that modify an active transaction.
- Parameters
-
[in] app A pointer to an allocation application. [in] name The name for the transaction.
- Returns
- A pointer to the transaction.
◆ newrelic_start_segment()
newrelic_segment_t* newrelic_start_segment | ( | newrelic_txn_t * | transaction, |
const char * | name, | ||
const char * | category | ||
) |
Record the start of a custom segment in a transaction.
Given an active transaction this function creates a custom segment to be recorded as part of the transaction. A subsequent call to newrelic_end_segment() records the end of the segment.
- Parameters
-
[in] transaction An active transaction. [in] name The segment name. If NULL or an invalid name is passed, this defaults to "Unnamed segment". [in] category The segment category. If NULL or an invalid category is passed, this defaults to "Custom".
- Returns
- A pointer to a valid custom segment; NULL otherwise.
- Examples
- ex_custom.c, ex_segment.c, ex_simple.c, and ex_timing.c.
◆ newrelic_start_web_transaction()
newrelic_txn_t* newrelic_start_web_transaction | ( | newrelic_app_t * | app, |
const char * | name | ||
) |
Start a web based transaction.
Given an application pointer and transaction name, this function begins timing a new transaction. It returns a valid pointer to an active New Relic transaction, newrelic_txn_t. The return value of this function may be used as an input parameter to functions that modify an active transaction.
- Parameters
-
[in] app A pointer to an allocation application. [in] name The name for the transaction.
- Returns
- A pointer to the transaction.
◆ newrelic_version()
const char* newrelic_version | ( | void | ) |
Get the SDK version.
- Returns
- A NULL-terminated string containing the C SDK version number. If the version number is unavailable, the string "NEWRELIC_VERSION" will be returned.
- Warning
- This string is owned by the SDK, and must not be freed or modified.
Generated on Wed Jan 8 2020 01:11:45 for New Relic C SDK by
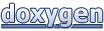