libnewrelic.h
newrelic_transaction_tracer_threshold_t threshold
Whether to consider transactions for trace generation based on the apdex configuration or a specific ...
Definition: libnewrelic.h:209
bool enabled
Specifies whether or not span events are generated.
Definition: libnewrelic.h:338
struct _newrelic_txn_t newrelic_txn_t
A New Relic transaction.
Definition: libnewrelic.h:106
newrelic_tt_recordsql_t
Configuration values used to configure how SQL queries are recorded and reported to New Relic.
Definition: libnewrelic.h:147
Definition: libnewrelic.h:138
char * uri
The URI that was loaded; it cannot be NULL.
Definition: libnewrelic.h:518
bool newrelic_accept_distributed_trace_payload(newrelic_txn_t *transaction, const char *payload, const char *transport_type)
Accept a distributed trace payload.
Definition: libnewrelic.h:191
Segment configuration used to instrument external calls.
Definition: libnewrelic.h:511
char * newrelic_create_distributed_trace_payload_httpsafe(newrelic_txn_t *transaction, newrelic_segment_t *segment)
Create a distributed trace payload, an http-safe, base64-encoded string.
bool newrelic_init(const char *daemon_socket, int time_limit_ms)
Initialise the C SDK with non-default settings.
newrelic_txn_t * newrelic_start_web_transaction(newrelic_app_t *app, const char *name)
Start a web based transaction.
char * procedure
The procedure used to load the external resource.
Definition: libnewrelic.h:533
bool newrelic_add_attribute_long(newrelic_txn_t *transaction, const char *key, const long value)
Add a custom long attribute to a transaction.
Configuration used to describe application name, license key, as well as optional transaction tracer ...
Definition: libnewrelic.h:347
char * query
Optional. Specifies the database query that was sent to the server.
Definition: libnewrelic.h:507
newrelic_custom_event_t * newrelic_create_custom_event(const char *event_type)
Creates a custom event.
newrelic_txn_t * newrelic_start_non_web_transaction(newrelic_app_t *app, const char *name)
Start a non-web based transaction.
struct _nr_app_and_info_t newrelic_app_t
A New Relic application. Once an application configuration is created with newrelic_create_app_config...
Definition: libnewrelic.h:95
char * newrelic_create_distributed_trace_payload(newrelic_txn_t *transaction, newrelic_segment_t *segment)
Create a distributed trace payload.
bool newrelic_set_segment_timing(newrelic_segment_t *segment, newrelic_time_us_t start_time, newrelic_time_us_t duration)
Override the timing for the given segment.
bool newrelic_end_segment(newrelic_txn_t *transaction, newrelic_segment_t **segment_ptr)
Record the completion of a segment in a transaction.
bool newrelic_configure_log(const char *filename, newrelic_loglevel_t level)
Configure the C SDK's logging system.
char * database_name
Optional. Specifies the database name or number in use.
Definition: libnewrelic.h:494
newrelic_transaction_tracer_config_t transaction_tracer
Optional. The transaction tracer configuration.
Definition: libnewrelic.h:390
Definition: libnewrelic.h:135
Configuration used to configure transaction tracing.
Definition: libnewrelic.h:195
Definition: libnewrelic.h:162
newrelic_app_config_t * newrelic_create_app_config(const char *app_name, const char *license_key)
Create a populated application configuration.
bool newrelic_custom_event_add_attribute_long(newrelic_custom_event_t *event, const char *key, long value)
Adds a long key/value pair to the custom event's attributes.
bool newrelic_accept_distributed_trace_payload_httpsafe(newrelic_txn_t *transaction, const char *payload, const char *transport_type)
Accept a distributed trace payload, an http-safe, base64-encoded string.
newrelic_segment_t * newrelic_start_datastore_segment(newrelic_txn_t *transaction, const newrelic_datastore_segment_params_t *params)
Record the start of a datastore segment in a transaction.
bool newrelic_add_attribute_double(newrelic_txn_t *transaction, const char *key, const double value)
Add a custom double attribute to a transaction.
newrelic_app_t * newrelic_create_app(const newrelic_app_config_t *config, unsigned short timeout_ms)
Create an application.
Definition: libnewrelic.h:152
newrelic_segment_t * newrelic_start_external_segment(newrelic_txn_t *transaction, const newrelic_external_segment_params_t *params)
Start recording an external segment within a transaction.
Definition: libnewrelic.h:129
Definition: libnewrelic.h:185
char * product
Specifies the datastore type, e.g., "MySQL", to indicate that the segment represents a query against ...
Definition: libnewrelic.h:445
newrelic_time_us_t duration_us
If the SDK configuration threshold is set to NEWRELIC_THRESHOLD_IS_OVER_DURATION, this field specifie...
Definition: libnewrelic.h:218
newrelic_time_us_t threshold_us
Specify the threshold above which a datastore query is considered "slow", in microseconds.
Definition: libnewrelic.h:275
bool newrelic_set_segment_parent_root(newrelic_segment_t *segment)
Set the transaction's root as the parent for the given segment.
newrelic_span_event_config_t span_events
Optional. Span event configuration.
Definition: libnewrelic.h:417
bool database_name_reporting
Configuration which controls whether datastore database names are reported to New Relic.
Definition: libnewrelic.h:307
bool instance_reporting
Configuration which controls whether datastore instance names are reported to New Relic.
Definition: libnewrelic.h:295
bool newrelic_set_transaction_timing(newrelic_txn_t *transaction, newrelic_time_us_t start_time, newrelic_time_us_t duration)
Override the timing for the given transaction.
void newrelic_discard_custom_event(newrelic_custom_event_t **event)
Frees the memory for custom events created via the newrelic_create_custom_event function.
Segment configuration used to instrument calls to databases and object stores.
Definition: libnewrelic.h:425
newrelic_time_us_t stack_trace_threshold_us
Sets the threshold above which the New Relic SDK will record a stack trace for a transaction trace,...
Definition: libnewrelic.h:226
bool newrelic_record_custom_metric(newrelic_txn_t *transaction, const char *metric_name, double milliseconds)
Generate a custom metric.
Definition: libnewrelic.h:132
bool newrelic_ignore_transaction(newrelic_txn_t *transaction)
Ignore the current transaction.
char * library
The library used to load the external resource.
Definition: libnewrelic.h:542
newrelic_distributed_tracing_config_t distributed_tracing
Optional. Distributed tracing configuration.
Definition: libnewrelic.h:408
void newrelic_record_custom_event(newrelic_txn_t *transaction, newrelic_custom_event_t **event)
Records the custom event.
bool newrelic_destroy_app_config(newrelic_app_config_t **config)
Destroy the application configuration.
char * collection
Optional. Specifies the table or collection being used or queried against.
Definition: libnewrelic.h:456
Configuration used to configure how datastore segments are recorded in a transaction.
Definition: libnewrelic.h:284
char * host
Optional. Specifies the datahost host name.
Definition: libnewrelic.h:479
newrelic_loglevel_t log_level
Optional. Specifies the logfile's level of detail.
Definition: libnewrelic.h:381
newrelic_segment_t * newrelic_start_segment(newrelic_txn_t *transaction, const char *name, const char *category)
Record the start of a custom segment in a transaction.
struct _newrelic_segment_t newrelic_segment_t
A segment within a transaction.
Definition: libnewrelic.h:840
bool newrelic_custom_event_add_attribute_string(newrelic_custom_event_t *event, const char *key, const char *value)
Adds a string key/value pair to the custom event's attributes.
struct _newrelic_custom_event_t newrelic_custom_event_t
A Custom Event.
Definition: libnewrelic.h:851
bool newrelic_end_transaction(newrelic_txn_t **transaction_ptr)
End a transaction.
newrelic_transaction_tracer_threshold_t
Configuration values used to configure the behaviour of the transaction tracer.
Definition: libnewrelic.h:180
Configuration used for distributed tracing.
Definition: libnewrelic.h:315
uint64_t newrelic_time_us_t
A time, measured in microseconds.
Definition: libnewrelic.h:115
bool newrelic_add_attribute_string(newrelic_txn_t *transaction, const char *key, const char *value)
Add a custom string attribute to a transaction.
void newrelic_notice_error(newrelic_txn_t *transaction, int priority, const char *errmsg, const char *errclass)
Record an error in a transaction.
Definition: libnewrelic.h:171
bool newrelic_set_transaction_name(newrelic_txn_t *transaction, const char *transaction_name)
Set a transaction name.
char * operation
Optional. Specifies the operation being performed: for example, "select" for an SQL SELECT query,...
Definition: libnewrelic.h:469
char * port_path_or_id
Optional. Specifies the port or socket used to connect to the datastore.
Definition: libnewrelic.h:487
bool enabled
Specifies whether or not distributed tracing is enabled.
Definition: libnewrelic.h:323
newrelic_datastore_segment_config_t datastore_tracer
Optional. The datastore tracer configuration.
Definition: libnewrelic.h:399
bool newrelic_custom_event_add_attribute_int(newrelic_custom_event_t *event, const char *key, int value)
Adds an int key/value pair to the custom event's attributes.
bool enabled
Whether to enable transaction traces.
Definition: libnewrelic.h:201
bool newrelic_add_attribute_int(newrelic_txn_t *transaction, const char *key, const int value)
Add a custom integer attribute to a transaction.
bool newrelic_set_segment_parent(newrelic_segment_t *segment, newrelic_segment_t *parent)
Set the parent for the given segment.
bool newrelic_custom_event_add_attribute_double(newrelic_custom_event_t *event, const char *key, double value)
Adds a double key/value pair to the custom event's attributes.
newrelic_tt_recordsql_t record_sql
Controls the format of the sql put into transaction traces for supported sql-like products.
Definition: libnewrelic.h:265
Generated on Wed Jan 8 2020 01:11:45 for New Relic C SDK by
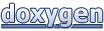